Visual Scripting Module 3: Understanding Variables and Data Types
Variables and data types are a crucial part of visual scripting and are universally applied across programming languages. A variable is a stored piece of data (like a name or number) that can be retrieved or changed by the program. Variables can hold a variety of different data types, and can either be retrieved (Get) or have their values assigned (Set).
Understanding Variables
Examine the following definitions:
Variable
- Definition: A variable is a storage location in your script that holds a value or a set of values. Variables can have their values retrieved or reassigned. Think of a variable as a labeled storage box that you can look at or change the contents of later.
- Use Cases: Variables can be used to store player scores, keep track of game states, or control animation states.
Set
- Definition: The set action allows you to assign a value to a variable. Think of it as putting something into the labeled storage box.
- Use Cases: Set can be used to assign initial values when a script starts, update values based on certain triggers or events, or reset values to their defaults.
Get
- Definition: The get action lets you retrieve the current value of a variable. Think of it as opening the storage box and looking at what's inside.
- Use Cases: Get can be used to check current game scores, determine if certain conditions are met, or use stored values to influence other parts of the script.
Data Type
- Definition: Every variable has a data type associated with it. The data type determines what kind of values the variable can hold. Common data types include numbers (integers or floats), booleans (true or false), strings (text), color, and more complex structures like lists or arrays. Picking the right data type is crucial, as using inappropriate data types can lead to errors, inefficient memory usage, or unexpected results.
Data Type | Description | Examples |
---|---|---|
Number | Represents either whole numbers (integers) or numbers with decimals (floats) | Integer: 1, 50, -23 Float: 1.23, 3.14, 0.91 |
Boolean | Represents only two possible values: true and false | True or False |
String | Represents a sequence of characters as text | Hello, Player1 |
Color | Represents a color value in HEX or RGB, with opacity | #1F00FF85 50%, (31, 0, 255) 21% |
Vec2 | Represents a point with two components, like a point in 2D space: (X, Y) | (1, 0) |
Vec3 | Represents a point with three components, like a point in 3D space: (X, Y, Z) | (2, -3, 0) |
Vec4 | Represents a point with four components: (X, Y, Z, W) | (-1, 0, 2, 1) |
Rect | Represents the dimensions of a rectangle | |
Texture 2D | Represents a 2D texture, often used for texture assets | Render Texture, Face Fusion Texture, or any imported texture |
Data Container
- Definition: Variables can contain either a single value, or a list of multiple values, called an array. The data container determines the variable as a single value or an array of values.
- Use Cases: A variable containing the string "Hello" uses a single data container. A variable with five different colors utilizes an array.
Get and Set Nodes and Properties
Get and Set are fundamental concepts in visual scripting that are relevant to nodes and properties in addition to variables.
There are nodes that will retrieve or set data according to a certain component or property. For example, Get Item from Array retrieves an item from an array, while Set Image sets the properties of the specified Image component.
Likewise, properties in the Inspector panel can be get or set, and can therefore be controlled through visual scripting. For example, take the Transform component. You can get or set its Position, Rotation, or Scale properties by clicking the circular button next to the property name.
Add Variables to the Visual Scripting Panel
To add a variable to the Visual Scripting panel, click the My Items button in the Visual Scripting toolbar. Then click the Add button [+] next to Variables in the My Items sidebar.
Click the Variable to view its details in the Details sidebar. Here you can customize the Name, Type (data type), Container (data container), and Default Value.
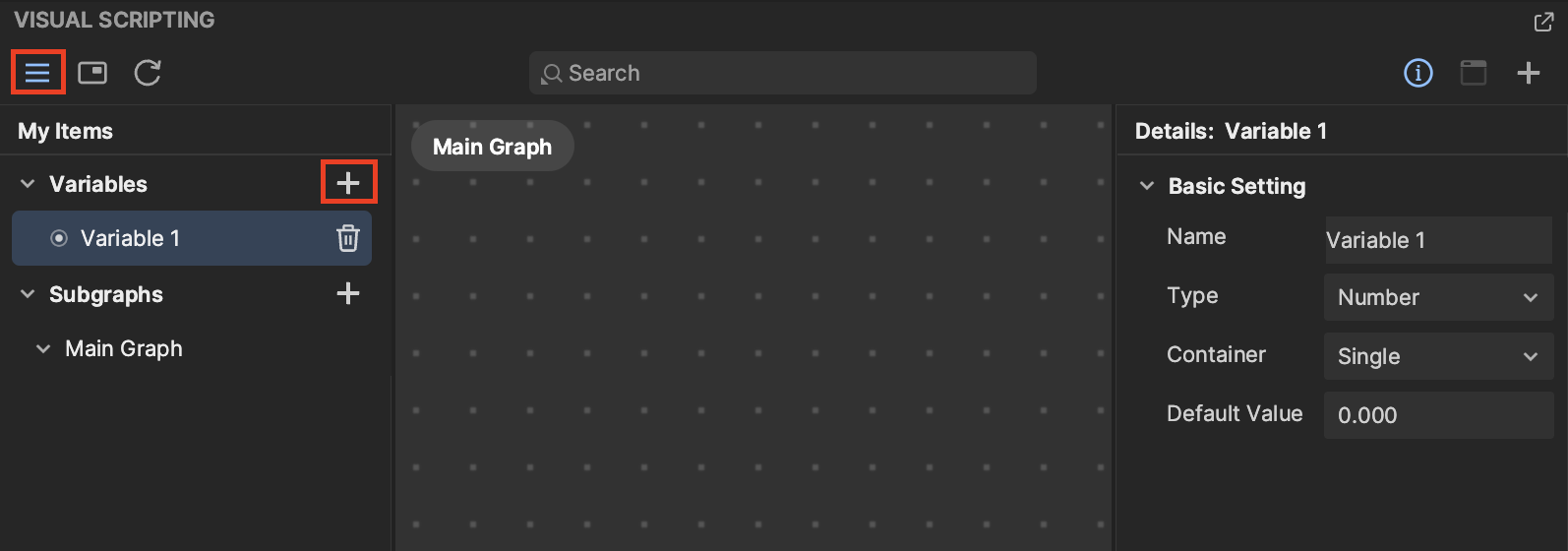
To add the variable as a node in the Visual Scripting panel, click the Create node button next to the variable's name. Then you can choose whether you want to Get variable or Set variable.
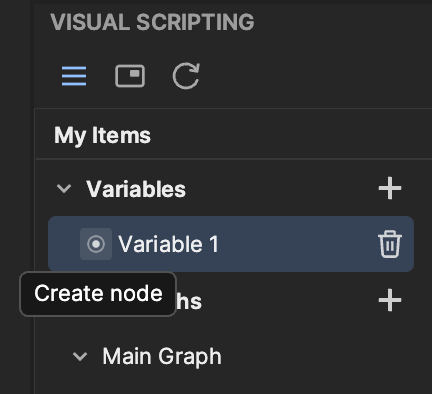
Variables Demo
Take a look at how you can use variables in visual scripting. First you'll learn how get and set can be applied to a single-valued number variable. Then you'll investigate how variables with arrays can be used.
The following examples are not necessarily complete flows of logic. Rather, they are examples of how variables can be used. Learn more about forming complete flows of logic in the next module.
Number Variable Example
Examine a number variable named Number. In the Details sidebar, notice that the Default Value is set to 10.000. You can set the Default Value to any desired number.
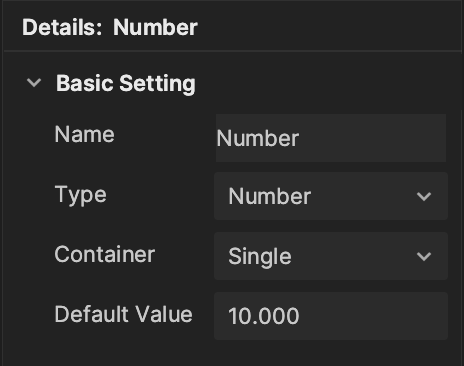
If a Default Value is not specified, it will be 0.000.
Take a look at the following snippet. The Number variable has been added to the Visual Scripting panel using both get and set.
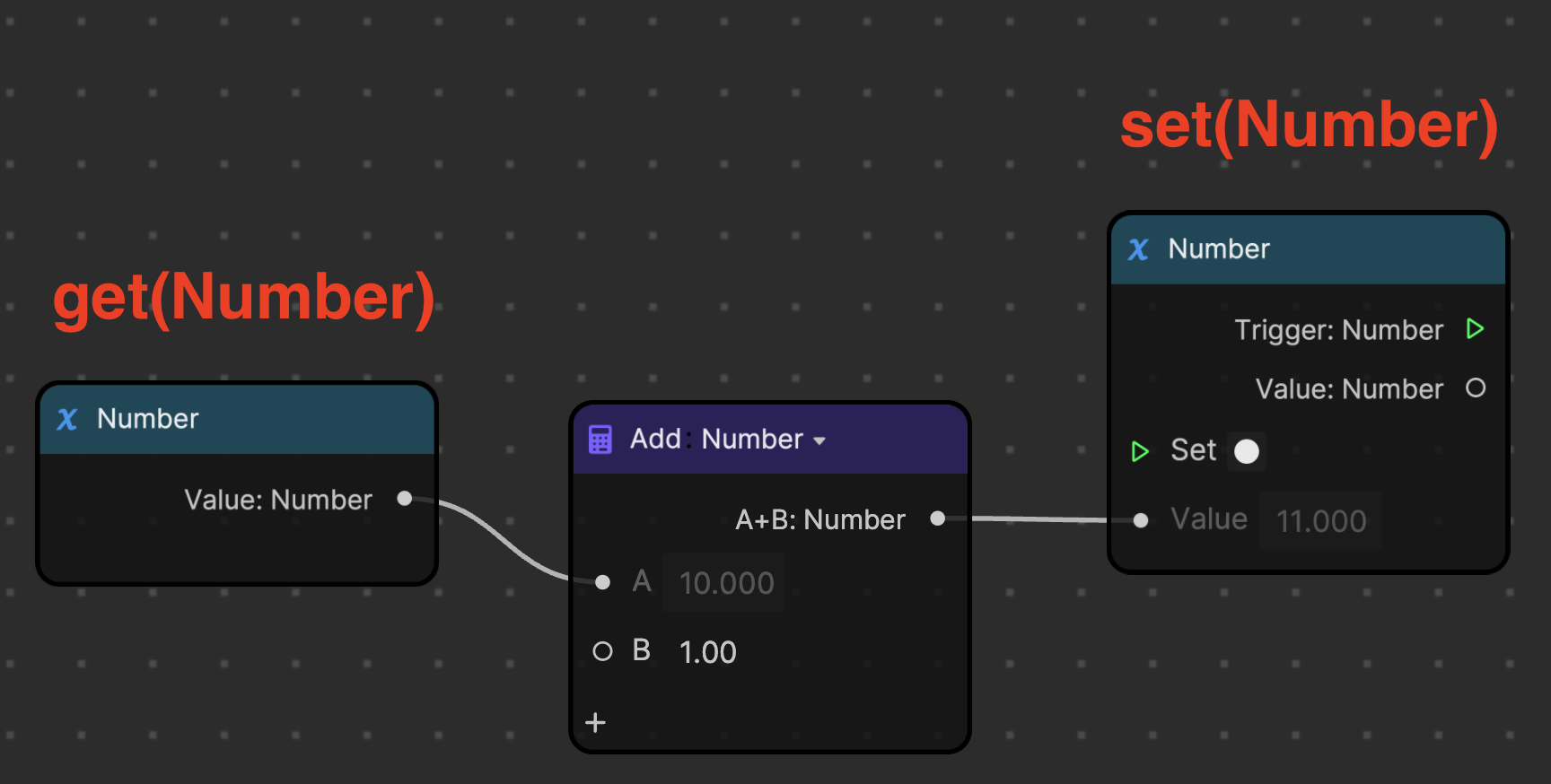
The get Number variable, whose value (10) is retrieved, inputs into the Add node, whose B input is set to 1.00. The Add node outputs the sum (11) into the set Number variable.
Recall that the set action assigns the value of a variable. In this case, the set Number variable should assign its input value, 11, as its own new value.
You can use the Peek node to check the value of the Number variable. Notice that the value is still 10.000.
The Peek node is useful for monitoring and checking values in real time.
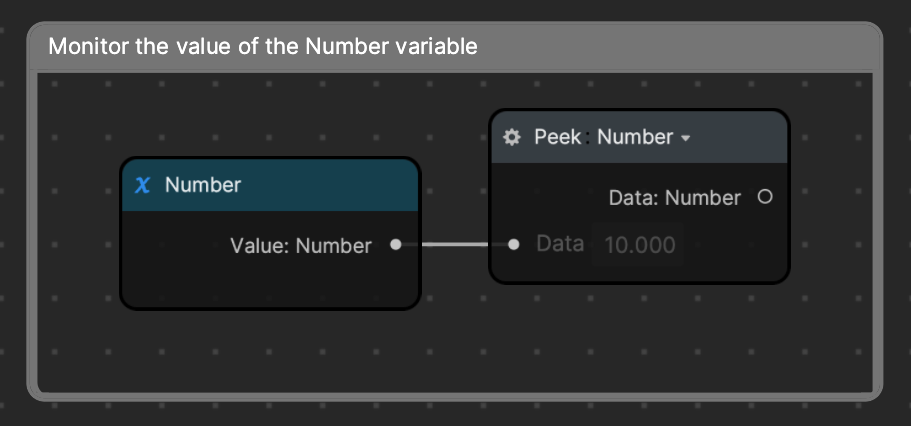
This is because Set variable only reassigns a value when a command is received from an upstream node. Therefore, you need something to tell the set Number variable to reassign the value to 11, like a node that detects screen taps.
Take note that Set variable only reassigns a value when a command is received. Learn more about the execution of commands in the next module.
Now examine the same snippet, with the Screen Tap node now added. The Screen Tap node, which commands the next node to perform its function when the screen is tapped, inputs into the set Number variable. It essentially tells the Number variable to reset its value every time the screen is tapped.
Notice that, every time the screen is tapped, the value of the Number variable changes to the previous value plus one, as displayed in the Peek node.
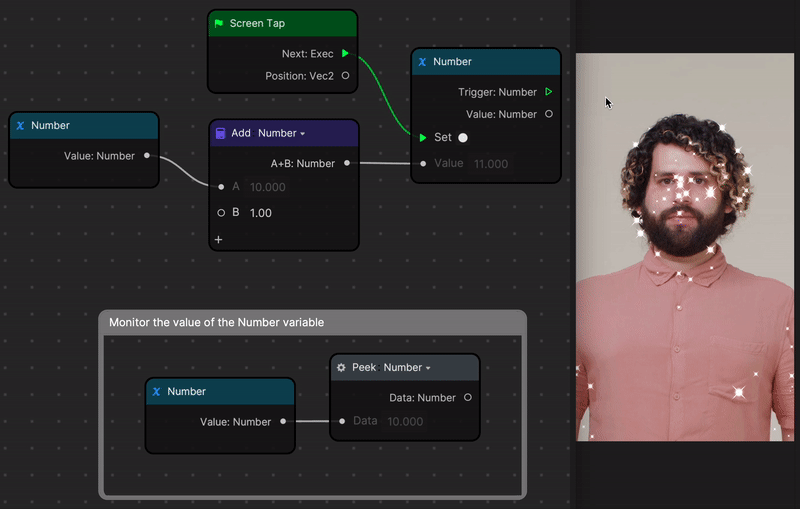
Variables in Interactive Game Effects
Now that you know how variables are used, take a look at how they can be implemented in game effects.
Side Scroll Jelly Game Template
This Side Scroll Jelly Game effect is a side-scrolling minigame that levitates a jellyfish every time you tap the screen. The objective of the game is to avoid obstacles and achieve a high score.
To open the Side Scroll Jelly Game template, go to the Templates tab on the home screen, click the Interactive tab, and then select Side Scroll Jelly Game.
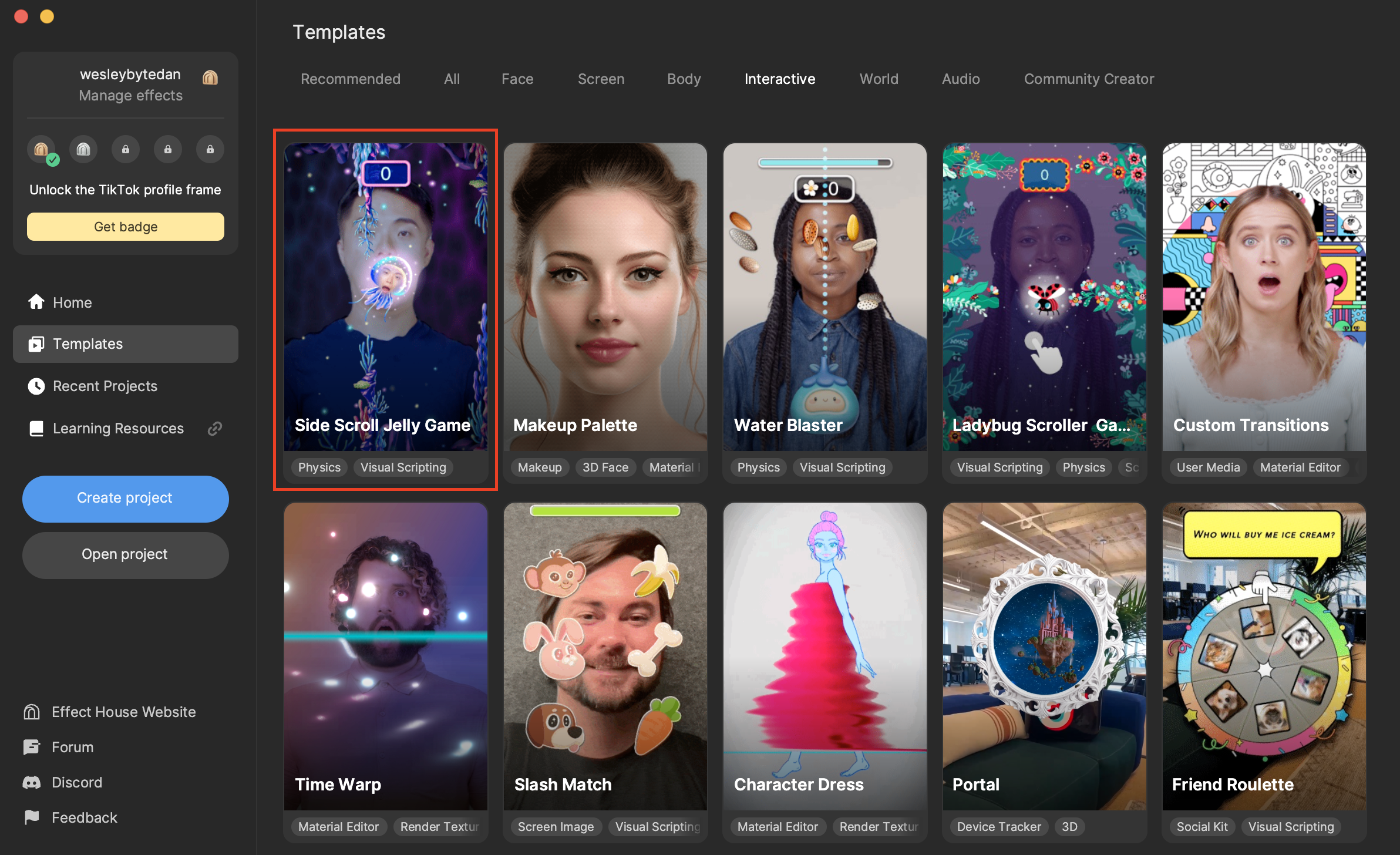
The effect requires multiple variables to be initialized before the game can start, like the scrolling speed of the background and foreground, the movement of decorative bubbles, and the tap force.
To view these initialized variables, double-click the Obstacle Avoidance Game subgraph, then double-click the underlying Initialize Variables subgraph. Notice how these variables use various Math nodes to set their initial values.
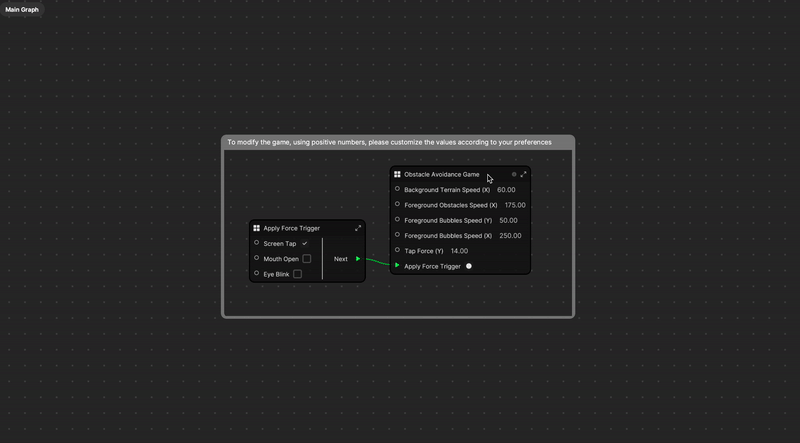
Key Terms
- Variable
- Get
- Set
- Data Type
- Number
- Boolean
- String
- Color
- Vec2
- Vec3
- Vec4
- Rect
- Texture2D
- Data Container
- Visual Scripting Nodes
- Add
- Peek
- Screen Tap
Next Steps
In the next module, you will apply your knowledge of nodes, variables, and data types to creating complete flows of logic in visual scripting.