Visual Scripting Module 6: Elevating Your Effects With Math
Math is a crucial part of visual scripting, and can be applied to create unique and dynamic logic flows. There are various Math nodes available in the Node menu.
In visual scripting, you'll often use variables in your math operations instead of static numbers. For example, when configuring a score for player health, you might set the variable health
as the difference between HP
and damage
: health = HP - damage
.
Math Nodes
Nodes in the Math category are organized alphabetically. The following sections group each node by operation type.
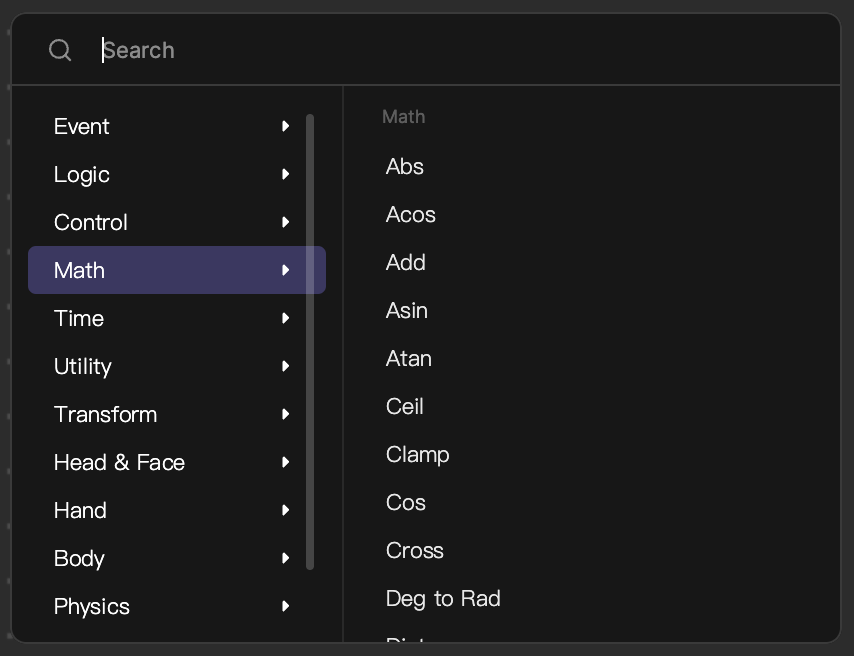
View the following sections for definitions and select examples of how math nodes are used.
Basic Arithmetic
Basic arithmetic nodes can be applied to simple computations, summations, averages, and ratios.
- Add: Calculates the sum of two inputs
- Subtract: Subtracts one value by another
- Multiply: Multiplies two numbers
- Divide: Divides one value by another
Rounding and Approximation
These nodes are used for approximation, graphics rendering, and discrete simulations.
- Ceil: Rounds up the input value to the nearest whole integer
- Floor: Rounds down the input value to the nearest whole integer
- Round: Rounds the input value to the nearest whole number
Advanced Arithmetic and Miscellaneous
The following nodes are often applied to simulate exponential growth, randomness in simulations, linear mapping, and curve fitting.
- Pow: Raises the input value A to the power of input B
- Sqrt: Calculates the square root of the input value
- Mod: Gives the remainder of input A divided by input B
In the Side Scroll Jelly Game template, Mod is used to update the number string, which shows up as the score.
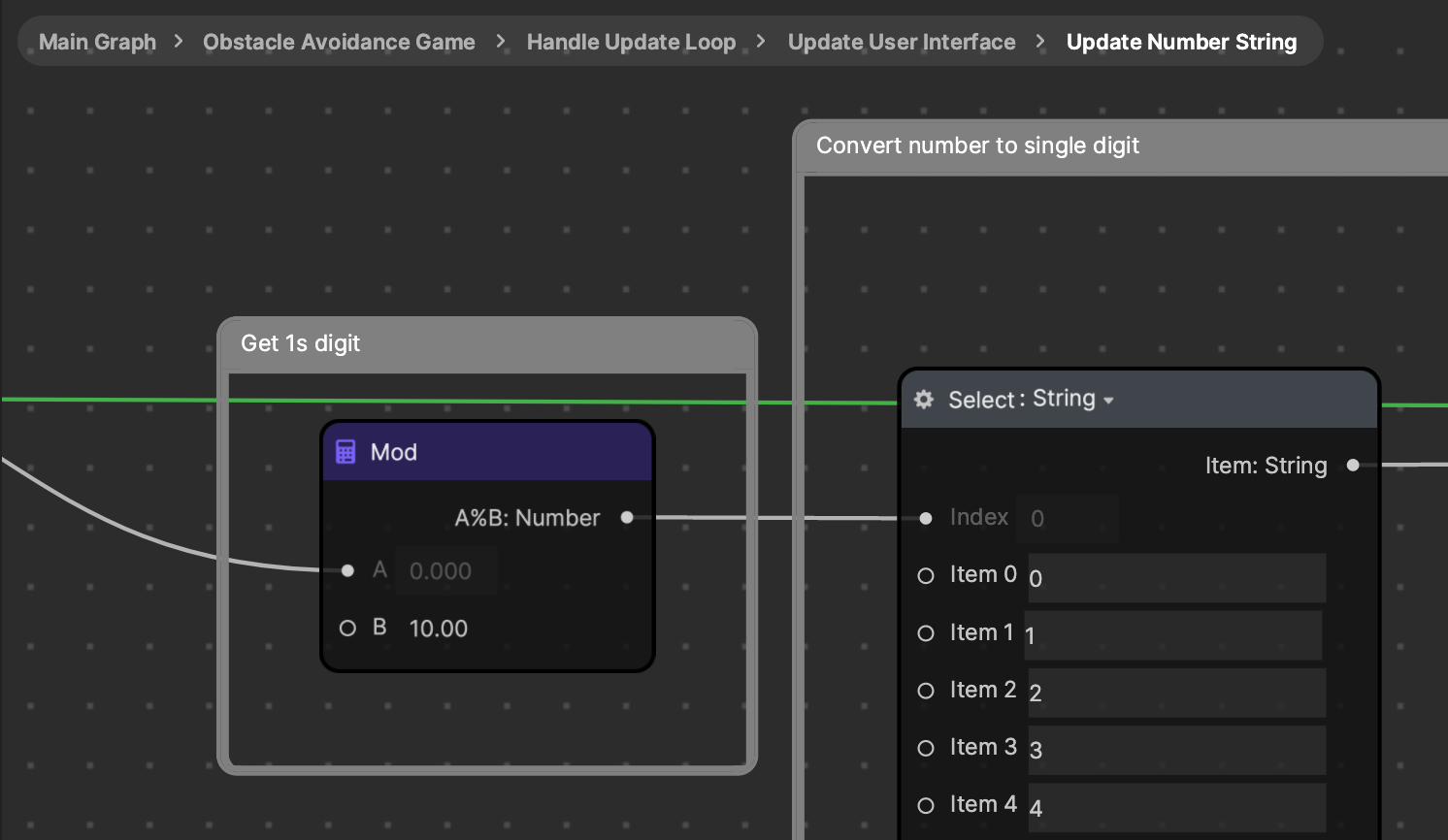
- Exp: Computes e, the base of natural logarithms raised to the power of input A
- Lerp: Linearly interpolates a value within a given range, where Start is the lower bound, Stop is the upper bound, and Step is the value used to interpolate between Start and Stop
Lerp is commonly used to create smooth transitions, like the reactive colors in the This or That template.
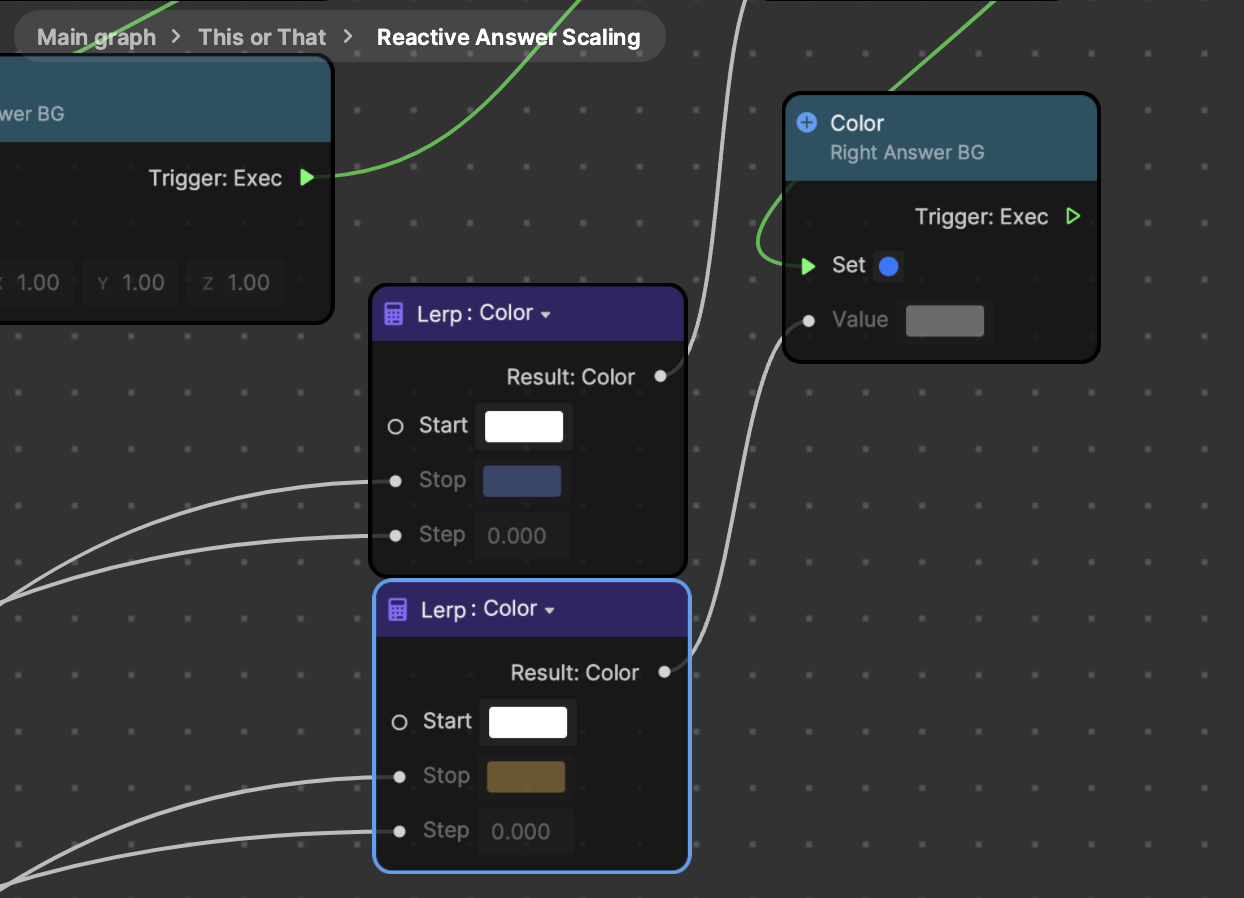
Clamp: Restricts a value to lie within a specified range. If the value is below the minimum of the range, then it is set to the minimum. If the value is above the maximum, then it is set to the maximum.
- For example, if you clamp the value 12 to the range [0, 10], the result would be 10 because 12 exceeds the maximum of the range.
Remap: Takes a value from one range and calculates its equivalent value in another range, maintaining the relative position of the value within the range.
- For example, if you remap a value of 5 from the range [0, 10] to the range [0, 100], the result would be 50 because 5 is at the midpoint of the original range, so its equivalent is at the midpoint of the new range.
The Side Scroll Jelly Game template uses Remap nodes to remap vertical velocity to rotation and animation speed.
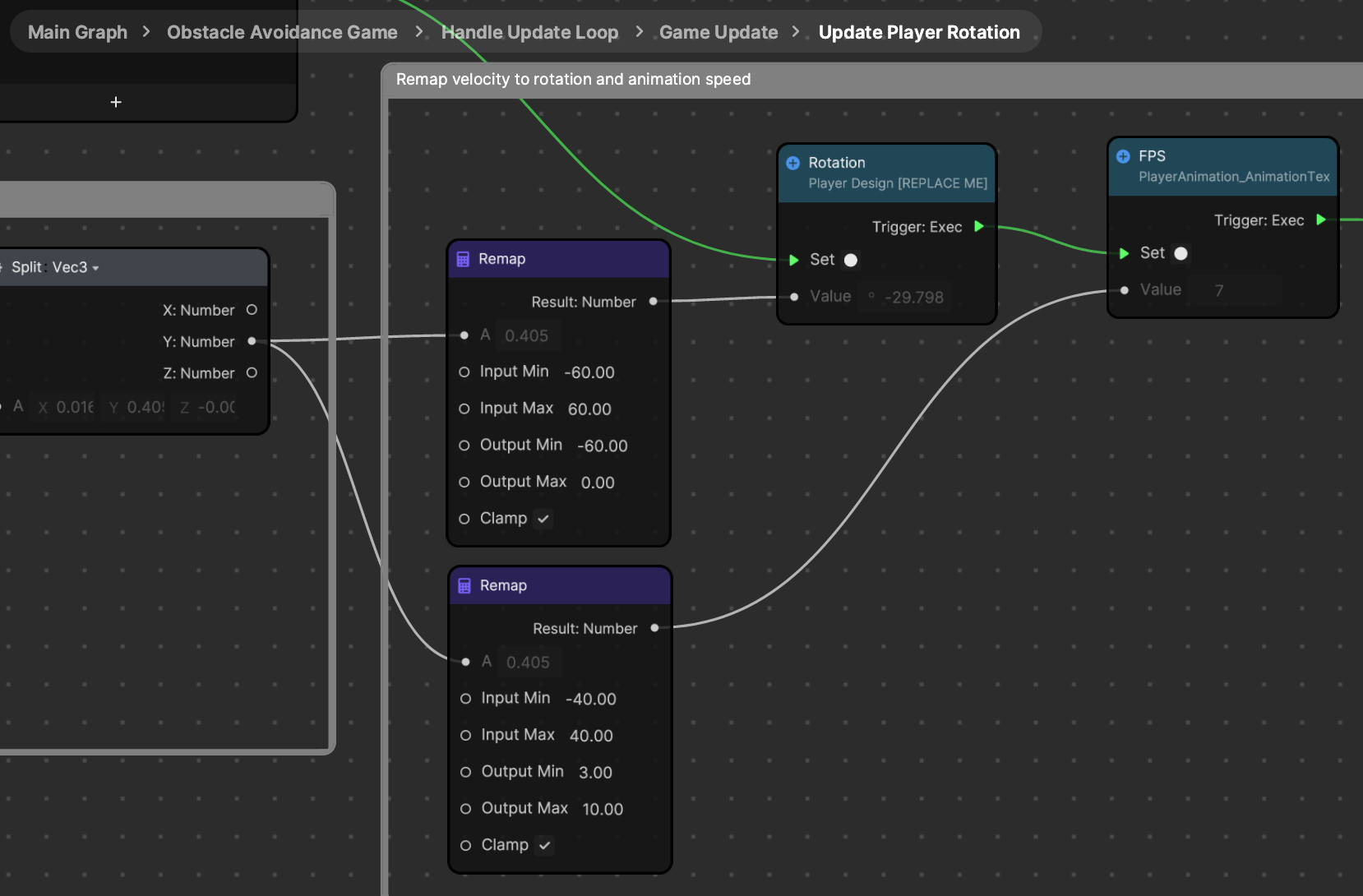
- Random: Generates a random number within a specified range.
The Friend Roulette template uses the Random node to determine how long the roulette wheel will spin for.
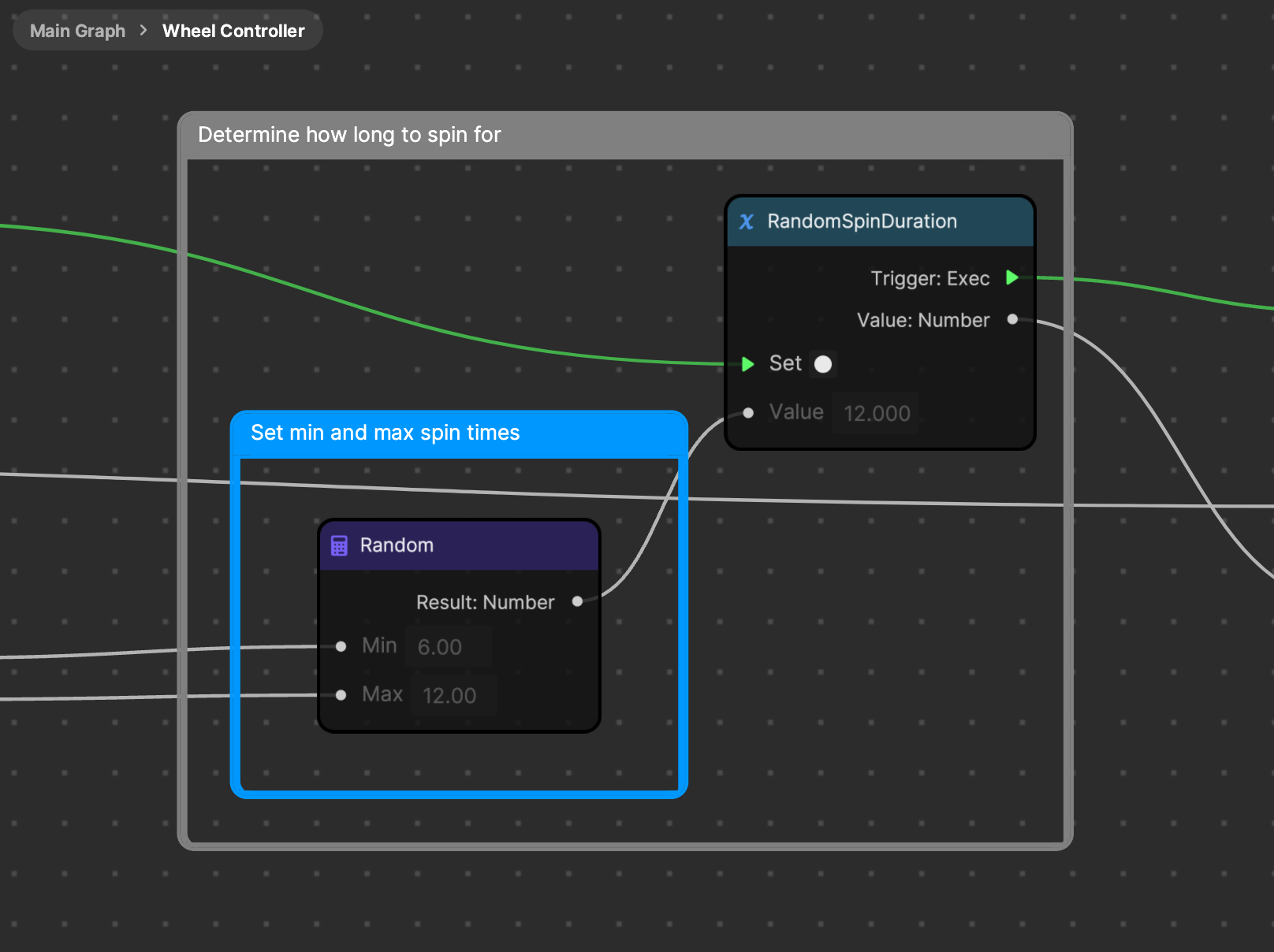
Value Evaluation
Evaluation nodes are commonly used in error correction, data validation, and range evaluations.
- Abs: Returns the absolute value of the input value.
- Max: Returns the greater of two input values.
- Min: Returns the lower of two input values.
Trigonometry
Trigonometry nodes are incorporated in rotation calculations, physics simulations, and geometric transformations.
- Acos: Calculates the arccosine value in radians
- Asin: Calculates the arcsine value in radians
- Atan: Calculates the arctangent value in radians
- Cos: Calculates the cosine value in radians
- Sin: Calculates the sine value in radians
- Deg to Rad: Converts angles from degrees to radians
- Rad to Deg: Converts angles from radians to degrees The Randomizer3D template uses Deg to Rad, Cos, and Sin nodes to evenly arrange objects in a circular layout
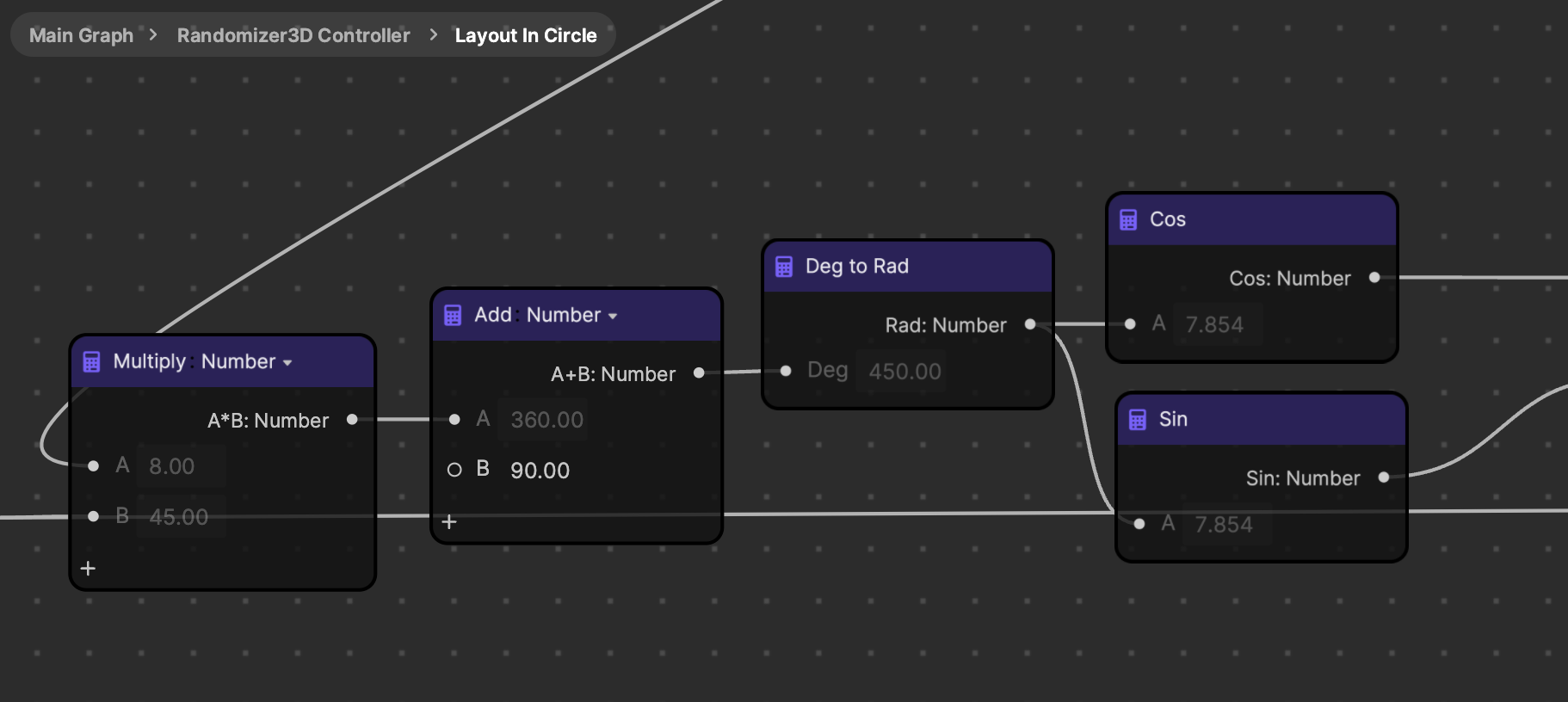
Vector Mathematics
Vector mathematics nodes are used in 3D graphics rendering, physics-based animations, and spatial computations.
- Normalize: Converts a vector to a unit vector
- Cross: Calculates the cross product of two vectors
- Dot: Determines the dot product of vectors
- Distance: Measures the magnitude between points
Math Node Example Applications
Inspect the following examples to see how select math nodes can be applied to certain scenarios.
Clamp: Ensures player health in a game remains within permissible limits
playerHealth = 110
minHealth = 0
maxHealth = 100
playerHealth = Clamp(playerHealth, minHealth, maxHealth)
// Result: playerHealth is now 100, as 110 is above the maximum permissible health of 100.
Remap: Changes the range of a volume slider from [0, 10] to [0, 100]
currentVolume = 5
originalRange = [0, 10]
newRange = [0, 100]
newVolume = Remap(currentVolume, originalRange, newRange)
// Result: newVolume is now 50.
Lerp: Gradually transitions between two colors over time
startColor = Red
endColor = Blue
transitionProgress = 0.5 // halfway
currentColor = Lerp(startColor, endColor, transitionProgress)
// Result: currentColor is a shade of purple, as it's halfway between red and blue.
Ceil: Calculates the number of boxes needed to pack items, where only whole boxes can be used
items = 7
itemsPerBox = 3
boxesNeeded = Ceil(items / itemsPerBox)
// Result: boxesNeeded is 3. Even though 7 items could fit into 2.33 boxes, you'd need 3 boxes.
Floor: Calculates whole hours from a given number of minutes
minutes = 125
hours = Floor(minutes / 60)
// Result: hours is 2. Because 125 minutes is equivalent to 2 hours and 5 minutes.
Round: Rounds off prices to the nearest dollar for a sale
price = 12.56
roundedPrice = Round(price)
// Result: roundedPrice is 13.
Mod: Determines if a number is even or odd
number = 15
remainder = number Mod 2
if remainder == 0:
// number is even
else:
// number is odd
// Result: 15 is odd.