Scoreboard
Welcome to MicroJam! We’ve created a subgraph that uses four Animation Sequence components on a 2D canvas to display a score, of up to 4 digits, in your effect! Just in case you need a refresher, subgraphs are a set of nodes that are grouped together to help better organize the Visual Scripting panel.
Start by downloading the following Effect House project and subgraph:
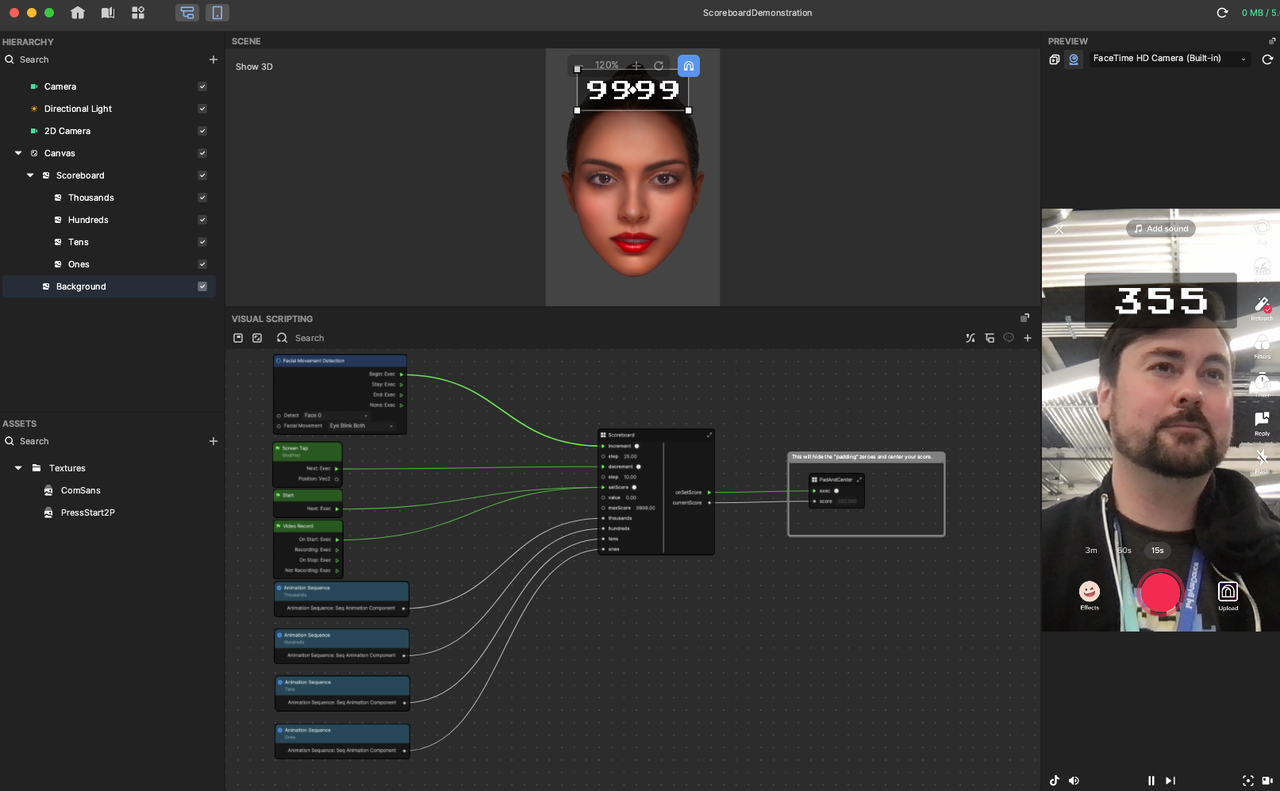
You can set, increase, and decrease the current score that your scoreboard displays using the three subgraph events. An event is denoted by the green triangle in between the input label and the green line that connects to it.
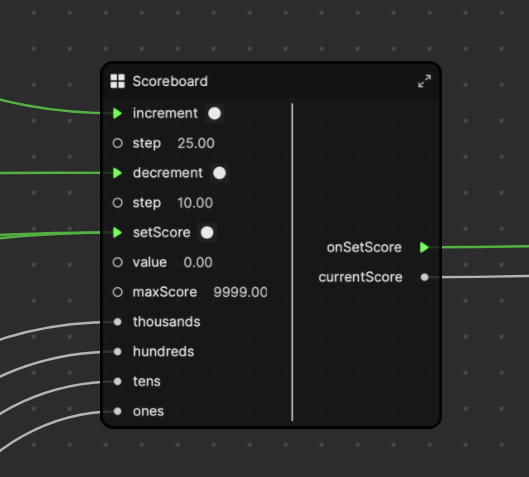
Hierarchy
This MicroJam project uses a single 2D camera with only one canvas, and everything is in one layer. Notice that there are four objects that represent the four digits of the score. The four objects are labeled based on which digit, or place, they represent in the score:
- Ones
- Tens
- Hundreds
- Thousands
The four objects are 2D images and each uses an Animation Sequence component to display a value between 0 and 9. We use Visual Scripting to control which number to set, instead of letting the Animation Sequence component loop continuously.
High-Level Visual Scripting Overview
The top-level Visual Scripting logic is almost entirely contained inside of the Scoreboard subgraph. If you take a look at the subgraph, you can see there are pairs of events that have an associated value. So if you connect an event like “when the user blinks” to increment, it will increment the score by the amount passed into step. Similarly, for decrement, you can reduce the score by the step value below it, and with setScore, you can hard set it to the value input.
To help you better understand which values are associated with the events above them, we’ve highlighted the related inputs in the following screenshot. Typically if you’re creating some sort of game or interaction, you can connect some related event to increment to increase the score. When you want the effect to reset, you can use setScore to reset it to 0.0.
To specify a maximum value other than 9999.00, you can use maxScore, a new addition to the subgraph.
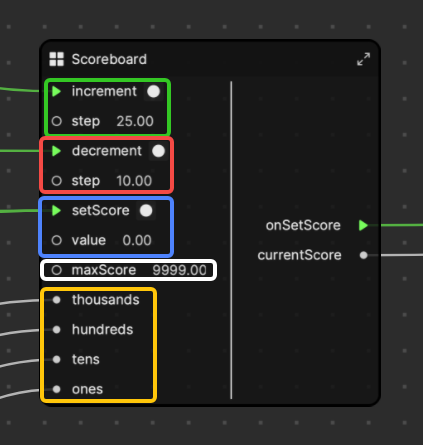
The yellow box highlights the Animation Sequence components that are being input. To create these nodes from scratch, find the Animation Sequence component of each digit, click the Pin-to-Graph button, and select Get Animation Sequence. If you use the provided Effect House project as the base, you can just use it as is.
Below you can see the various examples of connections we set up to demonstrate the simplest ways to use this subgraph.
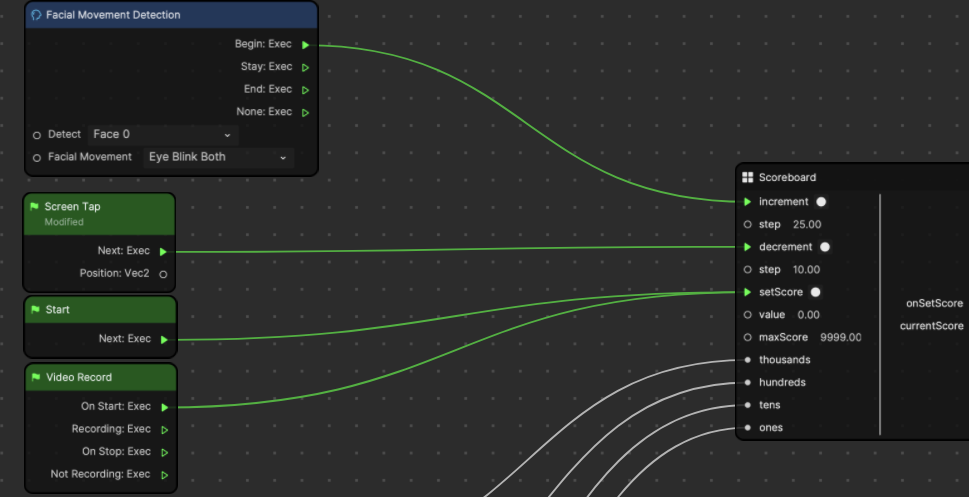
- The increment event is connected to the Begin event, which represents Eye Blink Both, the value of the Facial Movement event. This means that each time the user blinks, it will trigger the Facial Movement event and add 25.00 points to the score. The default incremental value for this subgraph is 25.00, but it can be set to your preferred value for your effect.
- The decrement event is connected to the Screen Tap node. This means that whenever the user taps on the screen during this event, the score will decrease by 10.00 points.
- The setScore event has two different events connected to it:
- One event comes from the Start node, which is triggered during the very first frame when the effect starts. This is a great way to set any values or initialize an effect that will also be reset later.
- The other event is the On Start event of the Video Record node, which resets the score to 0.00 when the user starts recording a video. This event resets the game, or interactive effect, each time the user begins recording.
As an experiment, you can try connecting the Next event and Delta Time value of the Update node to the increment event, as shown in the following screenshot.
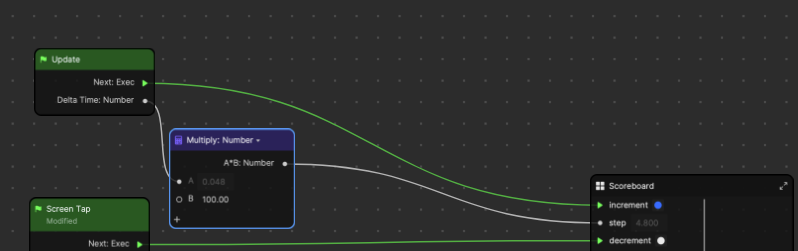
Lastly we’ve added a bonus subgraph to the updated scoreboard project that will adjust the placement and visibility of all the digits. If there is a single-digit number, this subgraph will hide the “000” before it. For instance, “0001” becomes “1” in this case.
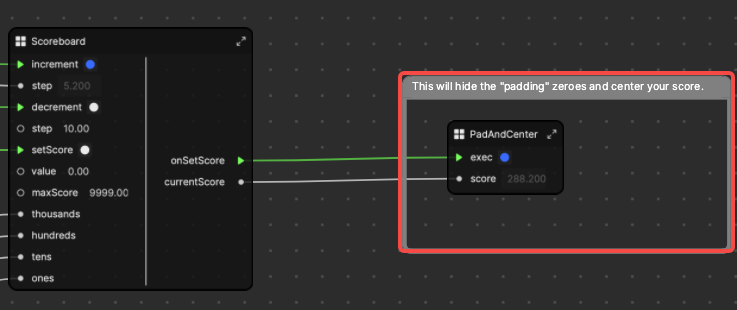
Disconnect and reconnect this subgraph to see the difference it makes.
Without bonus subgraph
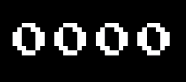
With bonus subgraph
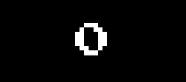
Deep Dive Into Visual Scripting
This Deep Dive Into Visual Scripting section is not necessary (and can be overkill) if you only want to use this MicroJam project as is. At this point, you already have the required knowledge to use this project. However, if you want to learn more about Visual Scripting and dive deeper into complex logic, please feel free to continue on. You are encouraged to recreate the following subgraph as you read through this section.
Control the Score
Notice in the screenshot below that there are three events, or actions, that can be done with this subgraph. As mentioned above, the three events are increment, decrement, and set. For this deep dive, we’ll highlight and explain how the nodes accomplish these actions.
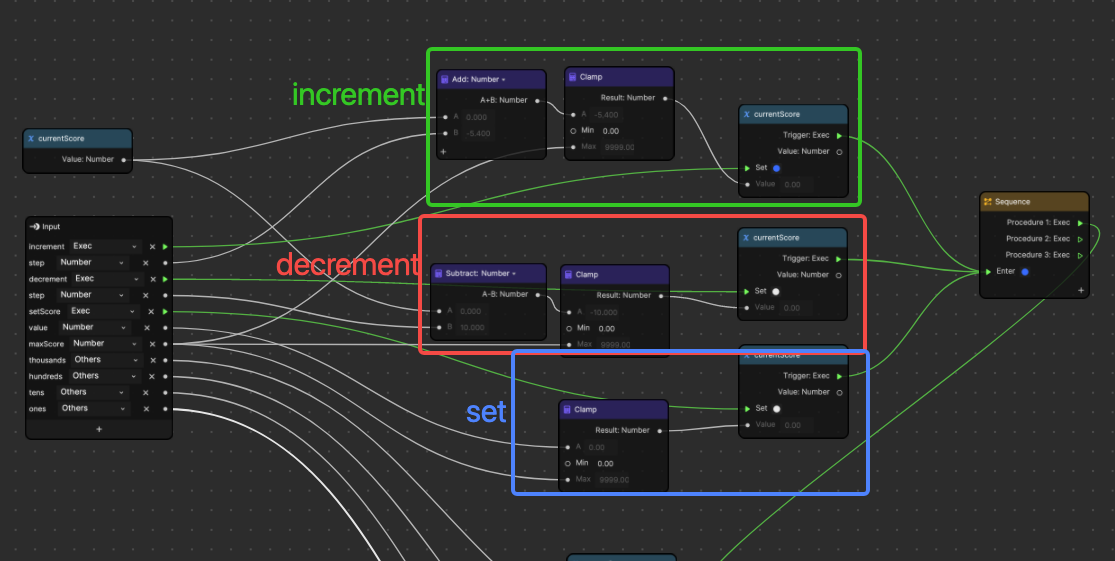
First notice that currentScore is saved to a variable so that we can keep track of it after the calculations are completed for every frame. We use currentScore and in the decrement and increment paths, we either add or subtract the input step values to calculate the new score.
You can also see that we use the Min and Max inputs to make sure that if the score is above our desired max score, or below 0, then it is clamped back to the defined limits, before it is set as the new value of the currentScore variable. The Set event is similar, except there are no mathematical calculations. Instead, we just simply set the score to the input value and then clamp it to the defined bounds.
Notice that all paths funnel into the same Sequence node. This is a good way to organize nodes if you need to make the connecting lines look a certain way. Really, you could omit this node and just directly connect all of the green lines directly to the next node, but then it would look messy. This is purely for organization, which is extremely important when working in the Visual Scripting panel. It’s easy to edit tidy subgraphs, but is difficult to edit a visually messy subgraph.
Display the Score
The second half of this subgraph will take in the updated currentScore and display the score to the screen by controlling the Animation Sequence components. Remember that our score is displayed using Image objects with Animation Sequence components attached to them. This allows us to set each digit to a value between 0 and 9.
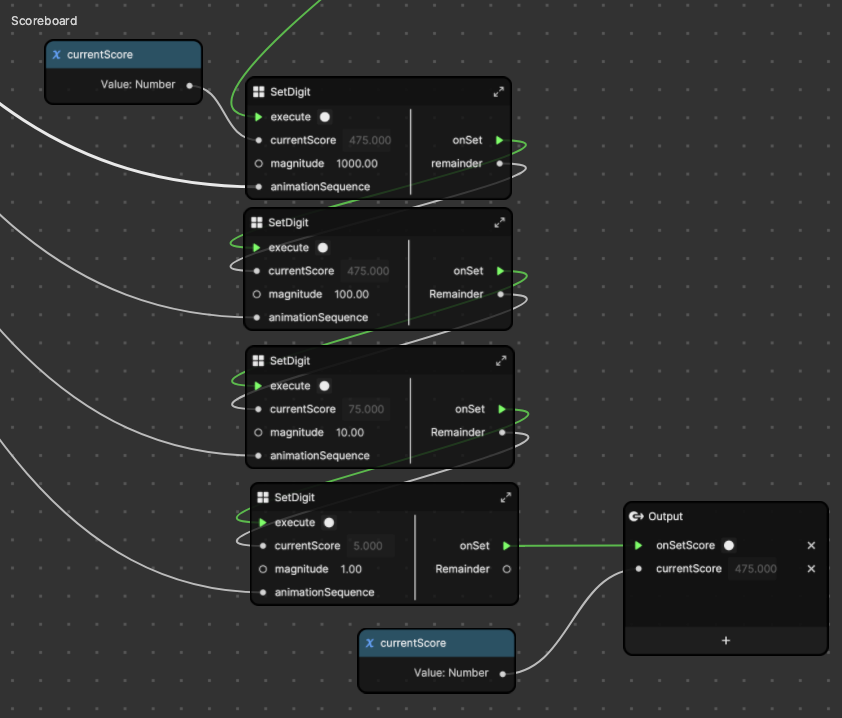
For each digit, we use math to extract the number that represents each digit. This is not an uncommon problem to solve in programming and there are a few different approaches you can use.
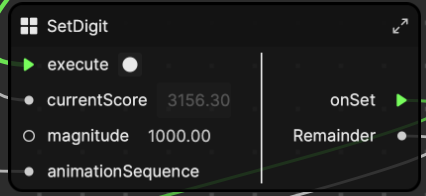
Let’s break down a SetDigit subgraph:
Parameter | Description |
---|---|
execute | The default trigger or event input. |
currentScore | The input number to process. |
magnitude | The magnitude of the digit we want to use (for example, 1000.00 uses the fourth digit, 1.00 uses the first digit, and so on). |
animationSequence | The reference to the animationSequence that is used to display this digit. |
onSet | The output trigger, so that the logic can continue on when this function is finished. |
Remainder | The remaining value after removing the digit. |
The overall flow looks like the following:
- Take in the value of the score.
- Do math to get only the left-most digit, which is decided by magnitude.
- Output the remaining value if that digit is removed.
- Pass the value of Remainder as the input to the next digit’s subgraph.
- Repeat steps 1 to 4 for four times, until all digits are removed and used.
- Inside each subgraph, the digit is also used to set animationSequence.
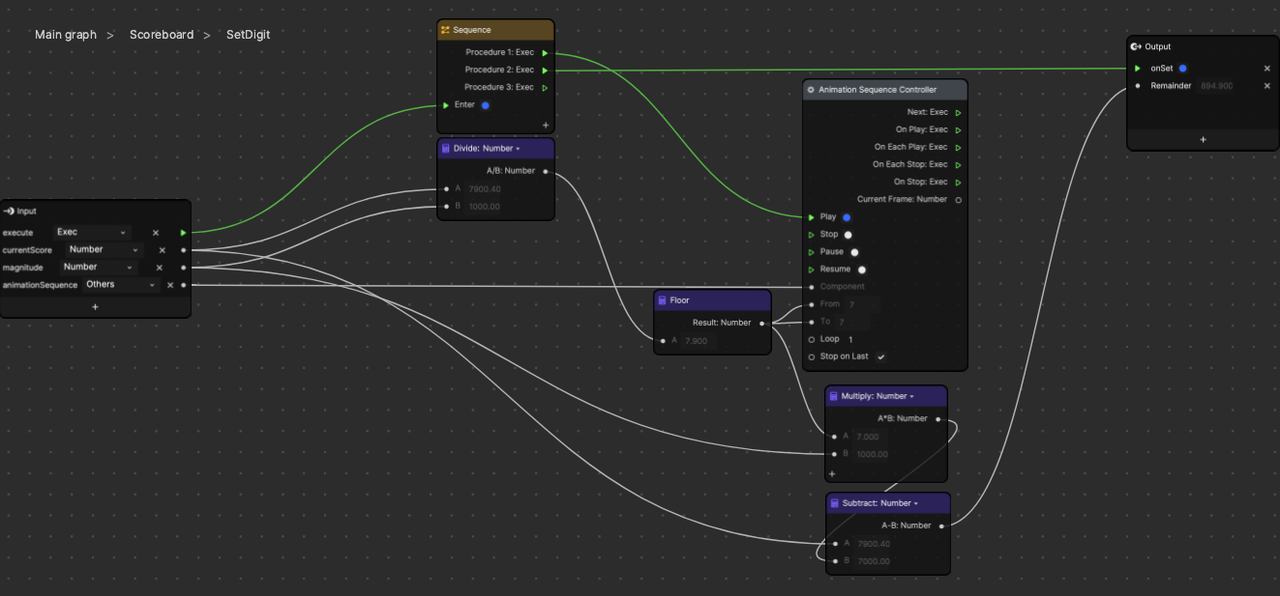
Looking at the SetDigit subgraph, we divide the input number by its magnitude. In this case, the mathematical calculation is 8778/1000, which is equal to 8.7. We then pass this value to the Floor node, which rounds down to 8. We want to display the digit, 8, for this subgraph.
We can pass 8 to the From and To input values of the Animation Sequence Controller node so that the animation sequence plays and only shows the 8th frame. This is one method that allows you to display a single image in an animation sequence with precise control.
If you take a look at the Remainder output, you can see that it is just taking the input value (8778.20) and subtracting the used value (8000.00) to give us the Remainder value to pass to the next SetDigit subgraph.
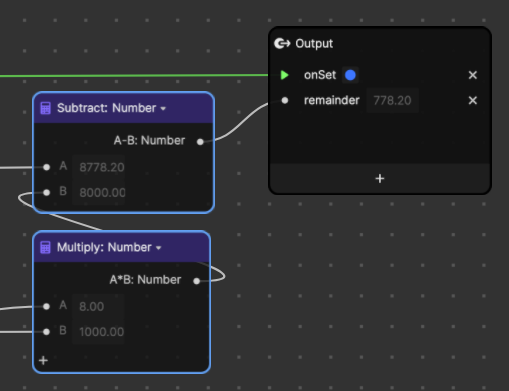
The last thing that happens after all the digits are set with these subgraphs is that we send an output signal from the Scoreboard subgraph, so that we can do things once the score is set. For instance, maybe you want to compare the current score to some maximum score and tell the player that they won when they’ve reached that score!
The following is an example of useful functions that will center the score and remove the “padding” zeros. This is a primary example of how you could use the output signal and output score!
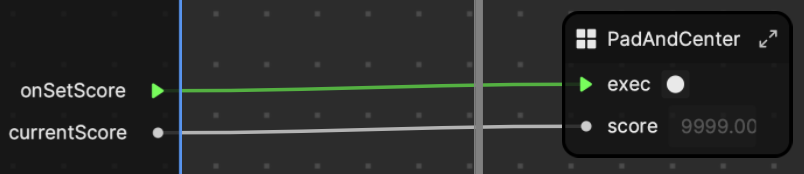
The PadAndCenter subgraph may look very large and industrial, but in reality, all it’s doing is checking the score’s magnitude, and then deciding which of the four presets to use. These presets have a horizontal offset and visibility value, which you can turn on and off, for each digit.
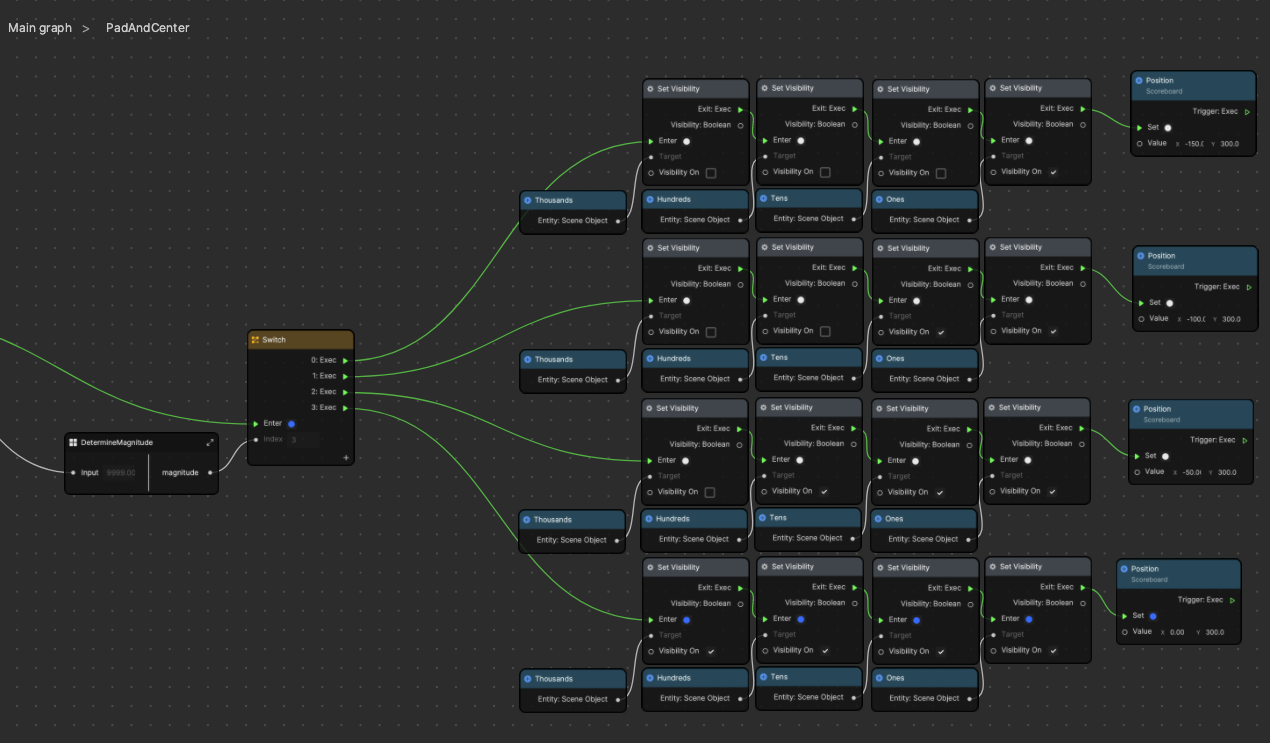
Bonus Quest: Negative Numbers Compatibility
The most basic version of this project is simple and doesn’t require a minus sign (-), which indicates a negative number. Adding a minus sign complicates the scoreboard, so it should only be added if there is a clear need for negative numbers. In the case that you require negative numbers, this section teaches you how to appropriately modify the Scoreboard subgraph.
Start by adding a Multiply node to the Scoreboard subgraph and multiplying maxScore by -1. This will give us the Min value, which is the same value as the max score.
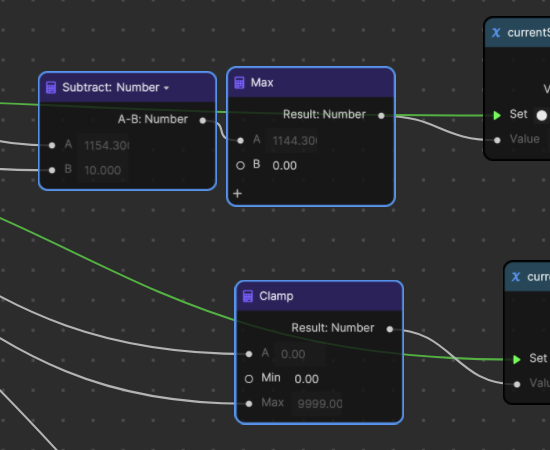
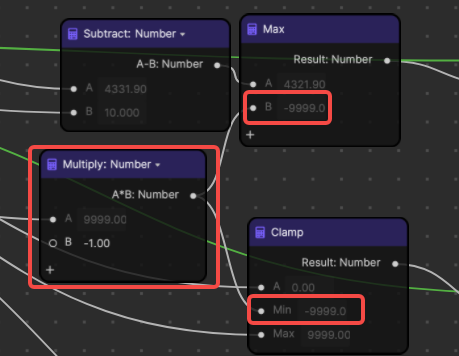
Next add an Abs (absolute value) node to process the current score before the digits are set.
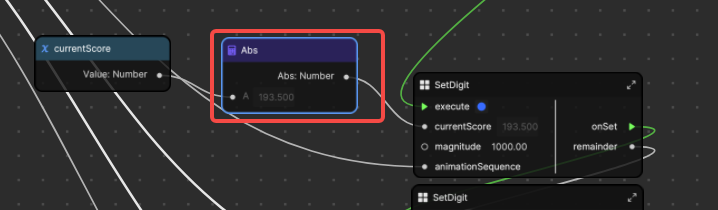
Lastly in the output of the subgraph, add some logic that enables or disables the minus sign, depending on whether the number is negative or positive. If you want to combine this with PadAndCenter, you’ll have to do a little more work, but that is a totally reasonable challenge for you to take on!
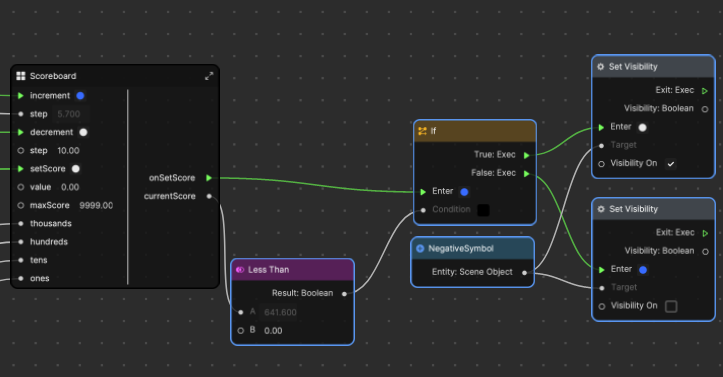
Fun Project Ideas
Now that we’ve completed this project, here are additional project ideas for you to experiment with:
- Create a game where a user must keep switching between opening their mouth and blinking their eyes to earn points. Challenge users to make the weirdest and funniest faces to compete for the highest score!
- Create a game where a random rotation is generated and a user must rotate their head by that many degrees. Points are awarded based on how close they are to the generated rotation value.
- Create a randomized “How funny are you?!” effect where a score displays a random number, in percentage, after a user tells a joke!
If you create anything with any of our MicroJam projects, please share it in the Effect House Discord channel and let us know what parts you used! We love receiving your feedback and seeing the amazing things you create.
Thanks for following along! Stay tuned for more MicroJam projects.